今日完成:
1、页面跳转
这块使用的是 presentViewController 模态弹出
在IOS开发中界面的跳转有三种方式:
1)改变Windows的根视图
2)利用UINavigationController进行push、pop
3)模态弹出
//忘记密码,弹出密码重置的页面
-(void)forget{
RepswViewController *repsw = [[RepswViewController alloc] init];
[self presentViewController:repsw animated:true completion:nil];
}
//点击注册,弹出注册账号的页面
-(void)sign{
SignViewController *signVC = [[SignViewController alloc] init];
[self presentViewController:signVC animated:false completion:nil];
}
2、“忘记密码?"和“注册”跳转后的两个页面
RepswViewController.m
//
// RepswViewController.m
// UIImageViewDemo
//
// Created by ptteng on 2019/10/21.
// Copyright © 2019 ptteng. All rights reserved.
//
#import "RepswViewController.h"
#import "CustomField.h"
@interface RepswViewController(){
CustomField * phoneField;
CustomField * codeView;
CustomField * newPswView;
UIButton * loginBtn;
UIButton * sentCode;
}
@end
@implementation RepswViewController
//收起键盘
-(void)touchesBegan:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event{
[self.view endEditing:YES];
}
- (instancetype)init
{
self = [super init];
if (self) {
self.modalPresentationStyle = UIModalPresentationFullScreen;
} return self;
}
-(void) viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor grayColor];
phoneField=[[CustomField alloc] init];
NSString *holderText = @"手机号码";
NSMutableAttributedString *placeholder = [[NSMutableAttributedString alloc] initWithString:holderText];
[placeholder addAttribute:NSForegroundColorAttributeName value:[UIColor whiteColor]
range:NSMakeRange(0,holderText.length)];
[placeholder addAttribute:NSFontAttributeName value:[UIFont systemFontOfSize:16]
range:NSMakeRange(0,holderText.length)];phoneField.attributedPlaceholder = placeholder;
[self.view addSubview:phoneField];
codeView= [[CustomField alloc] init];
NSString *holderText1 = @"输入验证码";
NSMutableAttributedString *placeholder1 = [[NSMutableAttributedString alloc] initWithString:holderText1];
[placeholder1 addAttribute:NSForegroundColorAttributeName value:[UIColor whiteColor]
range:NSMakeRange(0,holderText1.length)];
[placeholder1 addAttribute:NSFontAttributeName value:[UIFont systemFontOfSize:16]
range:NSMakeRange(0,holderText1.length)];codeView.attributedPlaceholder = placeholder1;
[self.view addSubview:codeView];
newPswView= [[CustomField alloc] init];
NSString *holderText2 = @"输入新密码";
NSMutableAttributedString *placeholder2 = [[NSMutableAttributedString alloc] initWithString:holderText2];
[placeholder2 addAttribute:NSForegroundColorAttributeName value:[UIColor whiteColor]
range:NSMakeRange(0,holderText2.length)];
[placeholder2 addAttribute:NSFontAttributeName value:[UIFont systemFontOfSize:16]
range:NSMakeRange(0,holderText2.length)];newPswView.attributedPlaceholder = placeholder2;
[self.view addSubview:newPswView];
// 确认按钮
loginBtn = [UIButton buttonWithType:UIButtonTypeSystem];
loginBtn.backgroundColor=[UIColor redColor];
// 设置按钮边框圆角
loginBtn.layer.cornerRadius=5;
loginBtn.layer.masksToBounds=YES;
[loginBtn setTitle:@"确认"
forState:UIControlStateNormal];
[loginBtn addTarget:self
// 设置触发事件login
action:@selector(login)
forControlEvents:UIControlEventTouchUpInside];
[loginBtn setTintColor:[UIColor whiteColor]];
[self.view addSubview:loginBtn];
//发送验证
sentCode = [UIButton buttonWithType:UIButtonTypeCustom];
sentCode.backgroundColor=[UIColor grayColor];
// 设置按钮边框圆角
sentCode.layer.cornerRadius=5;
sentCode.layer.masksToBounds=YES;
sentCode.layer.borderColor = [UIColor whiteColor].CGColor;//设置边框颜色
sentCode.layer.borderWidth = 0.5f;//设置边框颜色
[sentCode setTitle:@"发送验证码"
forState:UIControlStateNormal];
[sentCode addTarget:self
// 设置触发事件login
action:@selector(login)
forControlEvents:UIControlEventTouchUpInside];
[sentCode setTintColor:[UIColor whiteColor]];
[self.view addSubview:sentCode];
phoneField.translatesAutoresizingMaskIntoConstraints=NO;
codeView.translatesAutoresizingMaskIntoConstraints=NO;
loginBtn.translatesAutoresizingMaskIntoConstraints=NO;
newPswView.translatesAutoresizingMaskIntoConstraints=NO;
sentCode.translatesAutoresizingMaskIntoConstraints=NO;
// 自动布局
//phoneField.top与superview.top的约束
NSLayoutConstraint *phoneFieldTopTOSuperviewTop = [NSLayoutConstraint constraintWithItem:phoneField attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeTop multiplier:1 constant:100];
//phoneField.left与superview.left的约束
NSLayoutConstraint *phoneFieldLeftTOSuperviewLeft = [NSLayoutConstraint constraintWithItem:phoneField attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeLeft multiplier:1 constant:30];
//phoneField.right与superview.right的约束
NSLayoutConstraint *phoneFieldRightTOSuperviewRight = [NSLayoutConstraint constraintWithItem:phoneField attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeRight multiplier:1 constant:-30];
//codeView.top与phoneField.bottom的约束
NSLayoutConstraint *passwdFieldTopTOPhoneFieldBottom = [NSLayoutConstraint constraintWithItem:codeView attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:phoneField attribute:NSLayoutAttributeBottom multiplier:1 constant:30];
//codeView.left与superView.left的约束
NSLayoutConstraint *passwdFieldLeftTOSuperViewLeft = [NSLayoutConstraint constraintWithItem:codeView attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeLeft multiplier:1 constant:30];
// //codeView.right与sentCode.left的约束
NSLayoutConstraint *codeViewRightTOSendCodeLeft = [NSLayoutConstraint constraintWithItem:codeView attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:sentCode attribute:NSLayoutAttributeLeft multiplier:1 constant:-5];
//loginBtn.top与newPswView.bottom的约束
NSLayoutConstraint *loginBtnTopToPasswdFieldBottom = [NSLayoutConstraint constraintWithItem:loginBtn attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:newPswView attribute:NSLayoutAttributeBottom multiplier:1 constant:30];
//loginBtn.left与superView.left的约束
NSLayoutConstraint *loginBtnLeftTOSuperViewLeft = [NSLayoutConstraint constraintWithItem:loginBtn attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeLeft multiplier:1 constant:30];
//loginBtn.right与superView.right的约束
NSLayoutConstraint *loginBtnRightTOSuperViewRight = [NSLayoutConstraint constraintWithItem:loginBtn attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeRight multiplier:1 constant:-30];
//newPswView.top与codeView.bottom的约束
NSLayoutConstraint *newPswViewTopToCodeViewBottom = [NSLayoutConstraint constraintWithItem:newPswView attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:codeView attribute:NSLayoutAttributeBottom multiplier:1 constant:30];
//
//
//newPswView.left与superView.left的约束
NSLayoutConstraint *newPswViewLeftToSuperViewLeft= [NSLayoutConstraint constraintWithItem:newPswView attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeLeft multiplier:1 constant:30];
//newPswView.right与superView.right的约束
NSLayoutConstraint *newPswViewRightToSuperViewRight= [NSLayoutConstraint constraintWithItem:newPswView attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeRight multiplier:1 constant:-30];
//sentCode.right与superView.right的约束
NSLayoutConstraint *sendCodeRightToSuperViewRight= [NSLayoutConstraint constraintWithItem:sentCode attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeRight multiplier:1 constant:-30];
//sendCode.centerY与codeView.centerY的约束
NSLayoutConstraint *sendCodeCenterYToCodeViewCenterY= [NSLayoutConstraint constraintWithItem:sentCode attribute:NSLayoutAttributeBottom relatedBy:NSLayoutRelationEqual toItem:codeView attribute:NSLayoutAttributeBottom multiplier:1 constant:0];
//sendCode.width与codeView.width的约束
NSLayoutConstraint *sendCodeWidthToCodeViewWidth= [NSLayoutConstraint constraintWithItem:sentCode attribute:NSLayoutAttributeWidth relatedBy:NSLayoutRelationEqual toItem:codeView attribute:NSLayoutAttributeWidth multiplier:1 constant:-80];
[self.view addConstraints:@[phoneFieldTopTOSuperviewTop,phoneFieldLeftTOSuperviewLeft,phoneFieldRightTOSuperviewRight,passwdFieldTopTOPhoneFieldBottom,passwdFieldLeftTOSuperViewLeft,loginBtnTopToPasswdFieldBottom,loginBtnLeftTOSuperViewLeft,loginBtnRightTOSuperViewRight,newPswViewTopToCodeViewBottom,newPswViewLeftToSuperViewLeft,newPswViewRightToSuperViewRight
,sendCodeRightToSuperViewRight,sendCodeCenterYToCodeViewCenterY,sendCodeWidthToCodeViewWidth
,codeViewRightTOSendCodeLeft
]];
[self.view layoutIfNeeded];
}
//确认前,先做校验
- (void)login{
if (phoneField.text.length == 0) {
UIAlertController *alertController = [UIAlertController alertControllerWithTitle:@"温馨提示" message:@"登录名不能为空" preferredStyle:UIAlertControllerStyleAlert];
UIAlertAction *action = [UIAlertAction actionWithTitle:@"好的" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action) {
}];
[alertController addAction:action];
[self presentViewController:alertController animated:YES completion:nil];
return;
}
if (codeView.text.length == 0) {
UIAlertController *alertController = [UIAlertController alertControllerWithTitle:@"温馨提示" message:@"密码不能为空" preferredStyle:UIAlertControllerStyleAlert];
UIAlertAction *action = [UIAlertAction actionWithTitle:@"好的" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action) {
}];
[alertController addAction:action];
[self presentViewController:alertController animated:YES completion:nil];
return;
}
UIAlertController *alertController = [UIAlertController alertControllerWithTitle:@"温馨提示" message:@"登录成功" preferredStyle:UIAlertControllerStyleAlert];
UIAlertAction *action = [UIAlertAction actionWithTitle:@"好的" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action) {
}];
[alertController addAction:action];
[self presentViewController:alertController animated:YES completion:nil];
}
@end
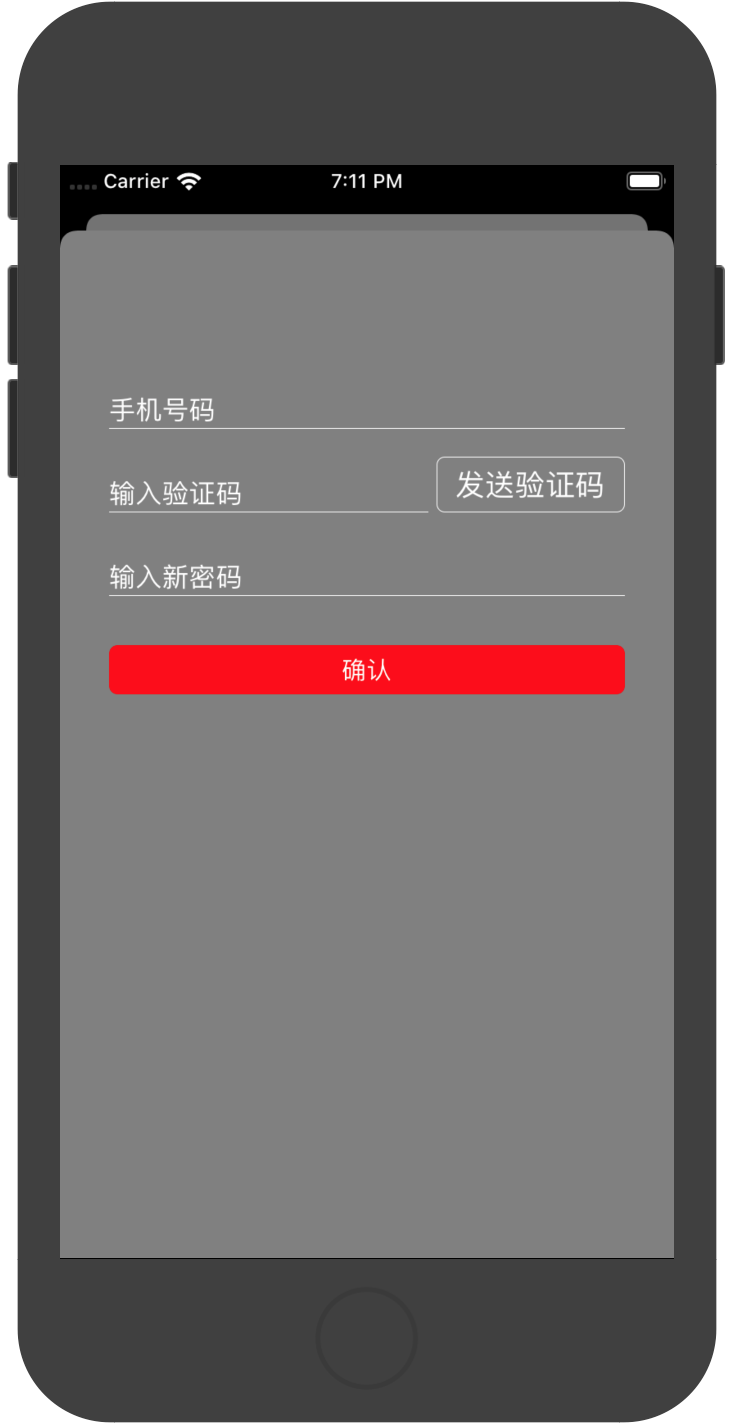
SignViewController.m
//
// SignViewController.m
// UIImageViewDemo
//
// Created by ptteng on 2019/10/21.
// Copyright © 2019 ptteng. All rights reserved.
//
#import "SignViewController.h"
#import "CustomField.h"
@interface SignViewController (){
CustomField * phoneField;
CustomField * codeView;
CustomField * newPswView;
UIButton * registerBtn;
UIButton * sendCodeView;
}
@end
@implementation SignViewController
//收起键盘
-(void)touchesBegan:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event{
[self.view endEditing:YES];
}
- (instancetype)init
{
self = [super init];
if (self) {
self.modalPresentationStyle = UIModalPresentationFullScreen;
} return self;
}
-(void) viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor grayColor];
phoneField=[[CustomField alloc] init];
NSString *holderText = @"手机号码";
NSMutableAttributedString *placeholder = [[NSMutableAttributedString alloc] initWithString:holderText];
[placeholder addAttribute:NSForegroundColorAttributeName value:[UIColor whiteColor]
range:NSMakeRange(0,holderText.length)];
[placeholder addAttribute:NSFontAttributeName value:[UIFont systemFontOfSize:16]
range:NSMakeRange(0,holderText.length)];phoneField.attributedPlaceholder = placeholder;
[self.view addSubview:phoneField];
codeView= [[CustomField alloc] init];
NSString *holderText1 = @"输入验证码";
NSMutableAttributedString *placeholder1 = [[NSMutableAttributedString alloc] initWithString:holderText1];
[placeholder1 addAttribute:NSForegroundColorAttributeName value:[UIColor whiteColor]
range:NSMakeRange(0,holderText1.length)];
[placeholder1 addAttribute:NSFontAttributeName value:[UIFont systemFontOfSize:16]
range:NSMakeRange(0,holderText1.length)];codeView.attributedPlaceholder = placeholder1;
[self.view addSubview:codeView];
newPswView= [[CustomField alloc] init];
NSString *holderText2 = @"密码";
NSMutableAttributedString *placeholder2 = [[NSMutableAttributedString alloc] initWithString:holderText2];
[placeholder2 addAttribute:NSForegroundColorAttributeName value:[UIColor whiteColor]
range:NSMakeRange(0,holderText2.length)];
[placeholder2 addAttribute:NSFontAttributeName value:[UIFont systemFontOfSize:16]
range:NSMakeRange(0,holderText2.length)];newPswView.attributedPlaceholder = placeholder2;
[self.view addSubview:newPswView];
// 确认按钮
registerBtn = [UIButton buttonWithType:UIButtonTypeSystem];
registerBtn.backgroundColor=[UIColor redColor];
// 设置按钮边框圆角
registerBtn.layer.cornerRadius=5;
registerBtn.layer.masksToBounds=YES;
[registerBtn setTitle:@"注册"
forState:UIControlStateNormal];
[registerBtn addTarget:self
// 设置触发事件login
action:@selector(Register)
forControlEvents:UIControlEventTouchUpInside];
[registerBtn setTintColor:[UIColor whiteColor]];
[self.view addSubview:registerBtn];
//发送验证
sendCodeView = [UIButton buttonWithType:UIButtonTypeCustom];
sendCodeView.backgroundColor=[UIColor grayColor];
// 设置按钮边框圆角
sendCodeView.layer.cornerRadius=5;
sendCodeView.layer.masksToBounds=YES;
sendCodeView.layer.borderColor = [UIColor whiteColor].CGColor;//设置边框颜色
sendCodeView.layer.borderWidth = 0.5f;//设置边框颜色
[sendCodeView setTitle:@"发送验证码"
forState:UIControlStateNormal];
[sendCodeView addTarget:self
// 设置触发事件login
action:@selector(sendCode)
forControlEvents:UIControlEventTouchUpInside];
[sendCodeView setTintColor:[UIColor whiteColor]];
[self.view addSubview:sendCodeView];
phoneField.translatesAutoresizingMaskIntoConstraints=NO;
codeView.translatesAutoresizingMaskIntoConstraints=NO;
registerBtn.translatesAutoresizingMaskIntoConstraints=NO;
newPswView.translatesAutoresizingMaskIntoConstraints=NO;
sendCodeView.translatesAutoresizingMaskIntoConstraints=NO;
// 自动布局
//phoneField.top与superview.top的约束
NSLayoutConstraint *phoneFieldTopTOSuperviewTop = [NSLayoutConstraint constraintWithItem:phoneField attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeTop multiplier:1 constant:100];
//phoneField.left与superview.left的约束
NSLayoutConstraint *phoneFieldLeftTOSuperviewLeft = [NSLayoutConstraint constraintWithItem:phoneField attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeLeft multiplier:1 constant:30];
//phoneField.right与superview.right的约束
NSLayoutConstraint *phoneFieldRightTOSuperviewRight = [NSLayoutConstraint constraintWithItem:phoneField attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeRight multiplier:1 constant:-30];
//codeView.top与phoneField.bottom的约束
NSLayoutConstraint *passwdFieldTopTOPhoneFieldBottom = [NSLayoutConstraint constraintWithItem:codeView attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:phoneField attribute:NSLayoutAttributeBottom multiplier:1 constant:30];
//codeView.left与superView.left的约束
NSLayoutConstraint *passwdFieldLeftTOSuperViewLeft = [NSLayoutConstraint constraintWithItem:codeView attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeLeft multiplier:1 constant:30];
// //codeView.right与sentCode.left的约束
NSLayoutConstraint *codeViewRightTOSendCodeLeft = [NSLayoutConstraint constraintWithItem:codeView attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:sendCodeView attribute:NSLayoutAttributeLeft multiplier:1 constant:-5];
//loginBtn.top与newPswView.bottom的约束
NSLayoutConstraint *loginBtnTopToPasswdFieldBottom = [NSLayoutConstraint constraintWithItem:registerBtn attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:newPswView attribute:NSLayoutAttributeBottom multiplier:1 constant:30];
//loginBtn.left与superView.left的约束
NSLayoutConstraint *loginBtnLeftTOSuperViewLeft = [NSLayoutConstraint constraintWithItem:registerBtn attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeLeft multiplier:1 constant:30];
//loginBtn.right与superView.right的约束
NSLayoutConstraint *loginBtnRightTOSuperViewRight = [NSLayoutConstraint constraintWithItem:registerBtn attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeRight multiplier:1 constant:-30];
//newPswView.top与codeView.bottom的约束
NSLayoutConstraint *newPswViewTopToCodeViewBottom = [NSLayoutConstraint constraintWithItem:newPswView attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:codeView attribute:NSLayoutAttributeBottom multiplier:1 constant:30];
//
//
//newPswView.left与superView.left的约束
NSLayoutConstraint *newPswViewLeftToSuperViewLeft= [NSLayoutConstraint constraintWithItem:newPswView attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeLeft multiplier:1 constant:30];
//newPswView.right与superView.right的约束
NSLayoutConstraint *newPswViewRightToSuperViewRight= [NSLayoutConstraint constraintWithItem:newPswView attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeRight multiplier:1 constant:-30];
//sentCode.right与superView.right的约束
NSLayoutConstraint *sendCodeRightToSuperViewRight= [NSLayoutConstraint constraintWithItem:sendCodeView attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:self.view attribute:NSLayoutAttributeRight multiplier:1 constant:-30];
//sendCode.centerY与codeView.centerY的约束
NSLayoutConstraint *sendCodeCenterYToCodeViewCenterY= [NSLayoutConstraint constraintWithItem:sendCodeView attribute:NSLayoutAttributeBottom relatedBy:NSLayoutRelationEqual toItem:codeView attribute:NSLayoutAttributeBottom multiplier:1 constant:0];
//sendCode.width与codeView.width的约束
NSLayoutConstraint *sendCodeWidthToCodeViewWidth= [NSLayoutConstraint constraintWithItem:sendCodeView attribute:NSLayoutAttributeWidth relatedBy:NSLayoutRelationEqual toItem:codeView attribute:NSLayoutAttributeWidth multiplier:1 constant:-80];
[self.view addConstraints:@[phoneFieldTopTOSuperviewTop,phoneFieldLeftTOSuperviewLeft,phoneFieldRightTOSuperviewRight,passwdFieldTopTOPhoneFieldBottom,passwdFieldLeftTOSuperViewLeft,loginBtnTopToPasswdFieldBottom,loginBtnLeftTOSuperViewLeft,loginBtnRightTOSuperViewRight,newPswViewTopToCodeViewBottom,newPswViewLeftToSuperViewLeft,newPswViewRightToSuperViewRight
,sendCodeRightToSuperViewRight,sendCodeCenterYToCodeViewCenterY,sendCodeWidthToCodeViewWidth
,codeViewRightTOSendCodeLeft
]];
[self.view layoutIfNeeded];
}
//发送验证码事件
- (void)sendCode{
if (phoneField.text.length == 0) {
UIAlertController *alertController = [UIAlertController alertControllerWithTitle:@"温馨提示" message:@"手机号码不能为空" preferredStyle:UIAlertControllerStyleAlert];
UIAlertAction *action = [UIAlertAction actionWithTitle:@"好的" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action) {
}];
[alertController addAction:action];
[self presentViewController:alertController animated:YES completion:nil];
return;
}
}
//注册事件
- (void)Register{
}
@end
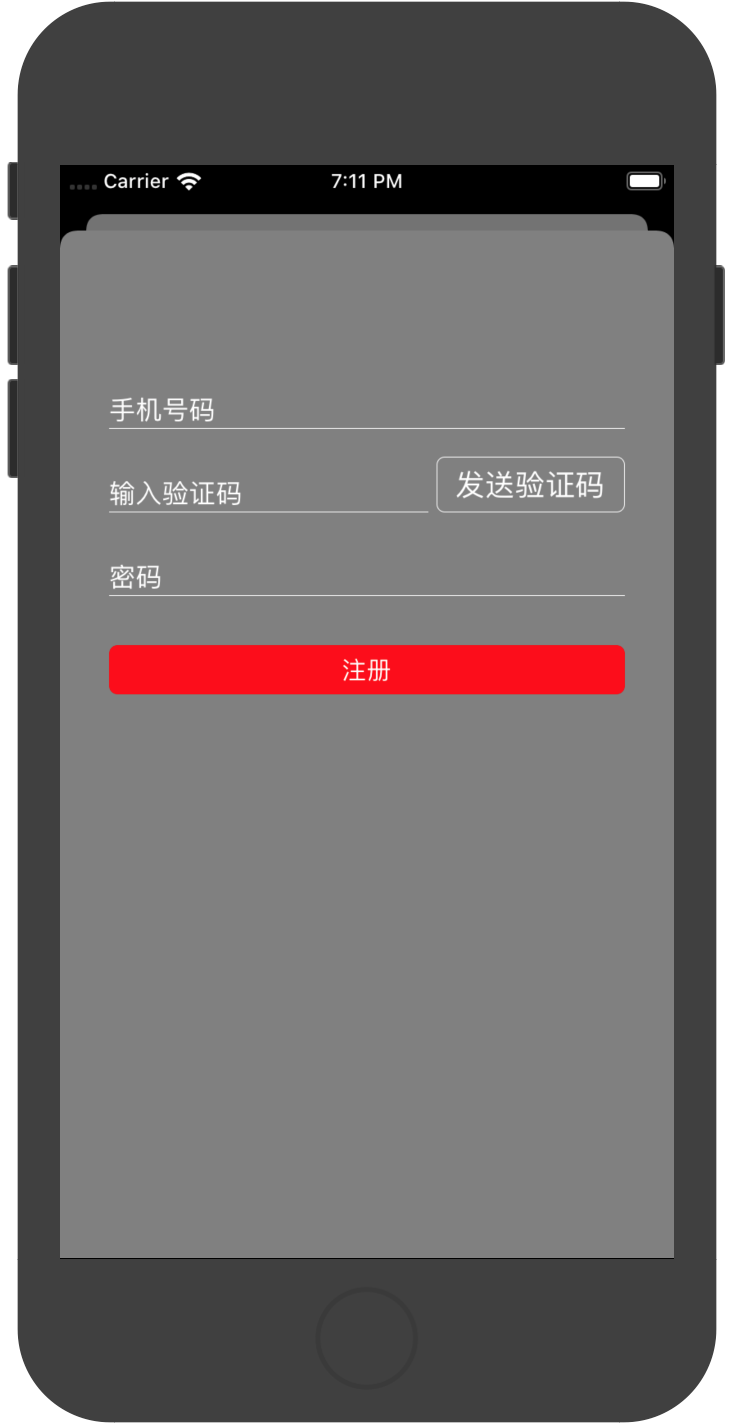
模态展示的页面不能全屏很不舒服,查了下,在iOS 13之前,模态展示的视图默认是全屏的。但更新到iOS13之后,默认的样式变成了iPhone上的Safari展示的分页样式。
解决方法:
在需要全屏展示的VC中添加如下代码:
- (instancetype)init
{
self = [super init];
if (self) {
self.modalPresentationStyle = UIModalPresentationFullScreen;
} return self;
}
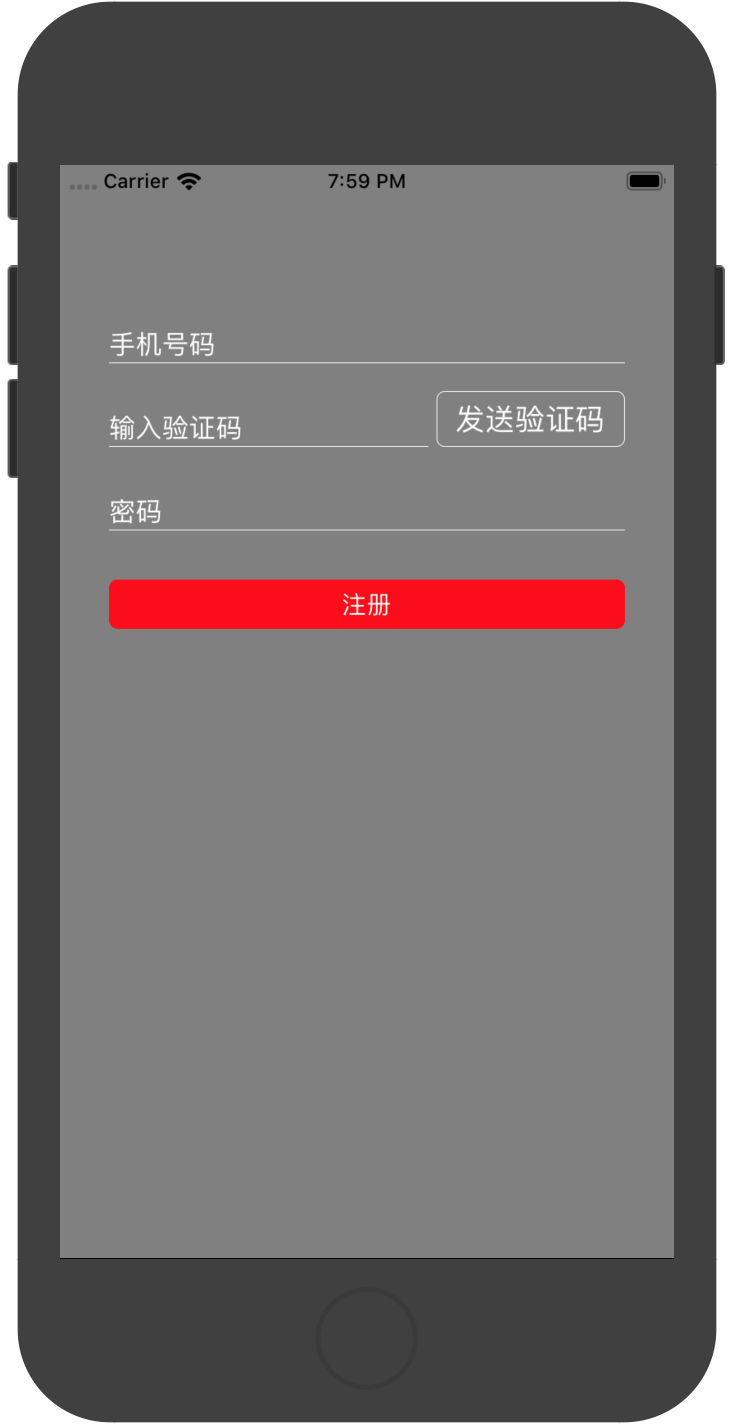
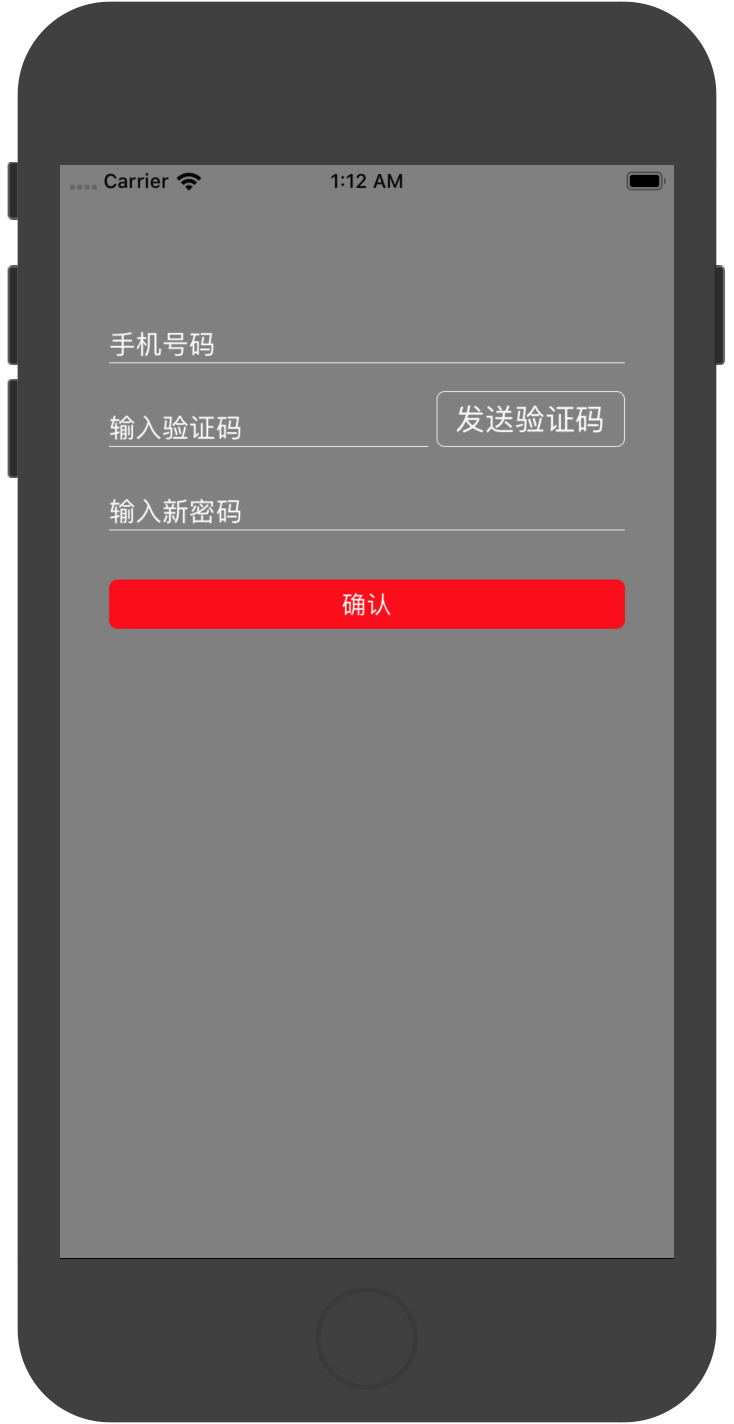
明日计划:
完成任务二的剩余部分:
向服务器发送请求
接收结果
用户名密码存储
遇到的问题:
这么点功能代码量咋这么大呢?
收获:
简单了解了下页面之间跳转的几种方式。
评论